Read a Part of Image Using Opencv
Reading, displaying, and writing images are basic to image processing and computer vision. Even when cropping, resizing, rotating, or applying different filters to process images, y'all'll need to offset read in the images. And so it's of import that yous chief these basic operations.
OpenCV, the largest figurer vision library in the world has these 3 congenital-in functions, let'due south observe out what exactly each 1 does:
-
imread()
helps u.s.a. read an epitome -
imshow()
displays an epitome in a window -
imwrite()
writes an prototype into the file directory
- Reading an Paradigm
- Displaying an Image
- Writing an Paradigm
- Summary
We will use the following prototype to demonstrate all the functions hither.
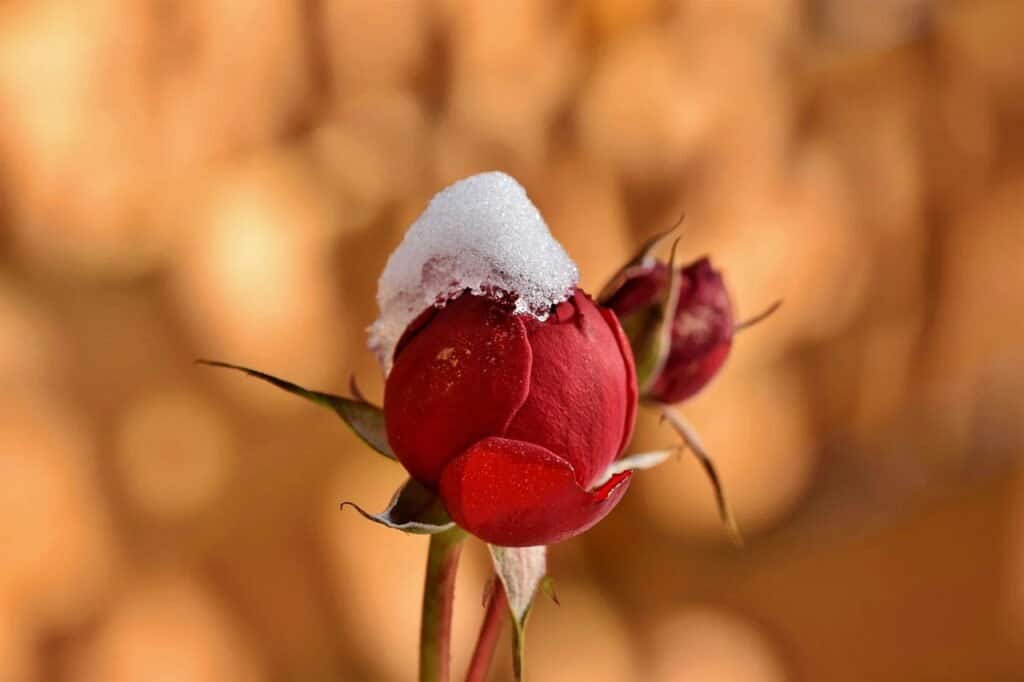
Starting time, get through this code example. Information technology reads and displays the in a higher place epitome. See, how information technology contains all the three functions, we but mentioned. As you lot continue further, we will discuss every single function used in this implementation.
Python
# import the cv2 library import cv2 # The part cv2.imread() is used to read an image. img_grayscale = cv2.imread('exam.jpg',0) # The function cv2.imshow() is used to display an epitome in a window. cv2.imshow('graycsale epitome',img_grayscale) # waitKey() waits for a primal press to shut the window and 0 specifies indefinite loop cv2.waitKey(0) # cv2.destroyAllWindows() simply destroys all the windows we created. cv2.destroyAllWindows() # The part cv2.imwrite() is used to write an epitome. cv2.imwrite('grayscale.jpg',img_grayscale)
Download Code To easily follow along this tutorial, please download code by clicking on the push beneath. It'southward FREE!
C++
//Include Libraries #include<opencv2/opencv.hpp> #include<iostream> // Namespace nullifies the apply of cv::function(); using namespace std; using namespace cv; // Read an image Mat img_grayscale = imread("test.jpg", 0); // Display the image. imshow("grayscale paradigm", img_grayscale); // Look for a keystroke. waitKey(0); // Destroys all the windows created destroyAllWindows(); // Write the image in the same directory imwrite("grayscale.jpg", img_grayscale);
Permit'due south brainstorm by importing the OpenCV library in Python and C++ (as shown beneath).
Python:
# import the cv2 library import cv2
In C++, utilize #include
(as shown beneath) to accomplish the same. Also specifying the namespaces for information technology lets y'all refer to role names directly. No need to prepend them with the namespace (e.m. instead of cv::imread()
, you can simply directly use read()
).
C++:
//Include Libraries #include<opencv2/opencv.hpp> #include<iostream> // Namespace nullifies the use of cv::function(); using namespace std; using namespace cv;
Reading an Image
For reading an image, use the imread()
part in OpenCV. Here'southward the syntax:
imread(filename, flags)
It takes two arguments:
- The start argument is the epitome name, which requires a fully qualified pathname to the file.
- The 2nd argument is an optional flag that lets yous specify how the image should be represented. OpenCV offers several options for this flag, but those that are well-nigh common include:
-
cv2.IMREAD_UNCHANGED
or-1
-
cv2.IMREAD_GRAYSCALE
or0
-
cv2.IMREAD_COLOR
or1
NEW COURSE Alive ON KICKSTARTER
Deep Learning With TensorFlow & Keras
The default value for flags is i, which will read in the epitome as a Colored image. When you desire to read in an paradigm in a particular format, but specify the appropriate flag. To check out the different flag options, click hither
It'due south likewise important to notation at this point that OpenCV reads color images in BGR format, whereas almost other reckoner vision libraries use the RGB channel format order. And then, when using OpenCV with other toolkits, don't forget to swap the bluish and carmine colour channels, equally you switch from one library to another.
As shown in the lawmaking sections below, nosotros will starting time read in the test image, using all three flag values described above.
Python
# Read an epitome img_color = cv2.imread('test.jpg',cv2.IMREAD_COLOR) img_grayscale = cv2.imread('test.jpg',cv2.IMREAD_GRAYSCALE) img_unchanged = cv2.imread('test.jpg',cv2.IMREAD_UNCHANGED)
C++
// Read an image Mat img_color = imread("test.jpg", IMREAD_COLOR); Mat img_grayscale = imread("test.jpg", IMREAD_GRAYSCALE); Mat img_unchanged = imread("test.jpg", IMREAD_UNCHANGED);
Or
Python
img_color = cv2.imread('test.jpg',i) img_grayscale = cv2.imread('test.jpg',0) img_unchanged = cv2.imread('test.jpg',-1)
C++
Mat img_color = imread("test.jpg", 1); Mat img_grayscale = imread("exam.jpg", 0); Mat img_unchanged = imread("test.jpg", -1);
Displaying an Image
In OpenCV, you lot display an image using the imshow()
role. Here's the syntax:
imshow(window_name, image)
This role also takes 2 arguments:
- The offset argument is the window proper name that volition be displayed on the window.
- The second argument is the paradigm that you want to display.
To display multiple images at once, specify a new window name for every image yous want to display.
The imshow()
function is designed to be used along with the waitKey()
and destroyAllWindows()
/ destroyWindow()
functions.
The waitKey()
part is a keyboard-binding office.
- It takes a single argument, which is the fourth dimension (in milliseconds), for which the window will be displayed.
- If the user presses whatever key within this time menstruum, the program continues.
- If 0 is passed, the program waits indefinitely for a keystroke.
- You tin besides set the function to detect specific keystrokes similar the Q key or the ESC cardinal on the keyboard, thereby telling more explicitly which fundamental shall trigger which beliefs.
The function destroyAllWindows()
destroys all the windows we created. If a specific window needs to be destroyed, give that verbal window name as the argument. Using destroyAllWindows()
also clears the window or paradigm from the master memory of the system.The code examples beneath show how the imshow()
office is used to display the images y'all read in.
Python
#Displays image within a window cv2.imshow('colour image',img_color) cv2.imshow('grayscale image',img_grayscale) cv2.imshow('unchanged image',img_unchanged) # Waits for a keystroke cv2.waitKey(0) # Destroys all the windows created cv2.destroyAllwindows()
C++
// Create a window. namedWindow( "colour image", WINDOW_AUTOSIZE ); namedWindow( "grayscale image", WINDOW_AUTOSIZE ); namedWindow( "unchanged image", WINDOW_AUTOSIZE ); // Show the paradigm within it. imshow( "colour image", img_color ); imshow( "grayscale image", img_grayscale ); imshow( "unchanged prototype", img_unchanged ); // Expect for a keystroke. waitKey(0); // Destroys all the windows created destroyAllWindows();
Beneath is the GIF demonstrating the process of executing the code, visualizing the outputs, and closing the output window:
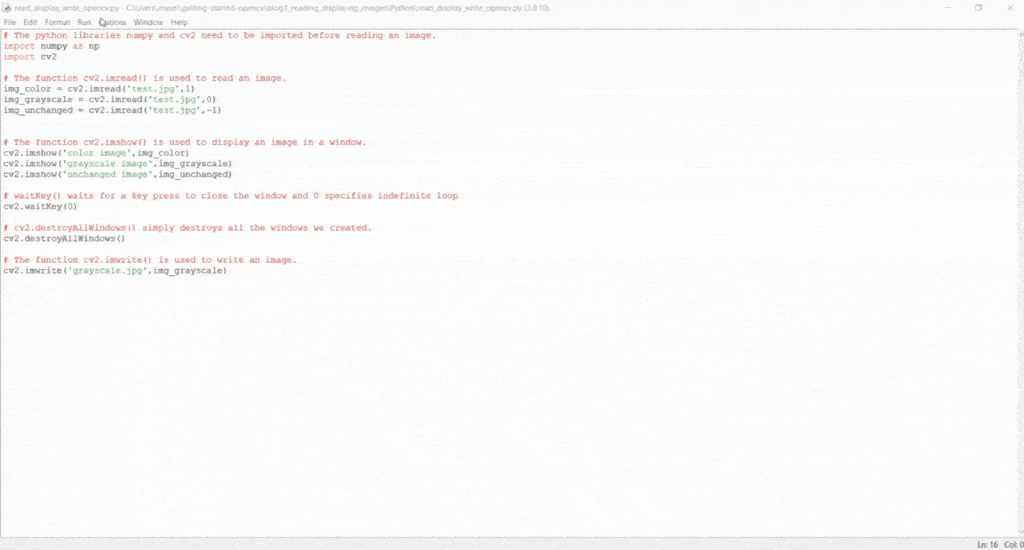
In the three output screens shown below, yous can see:
- The starting time epitome is displayed in colour
- The side by side as grayscale
- The tertiary is once more in color, equally this was the original format of the image (which was read using
cv2.IMREAD_UNCHANGED
)
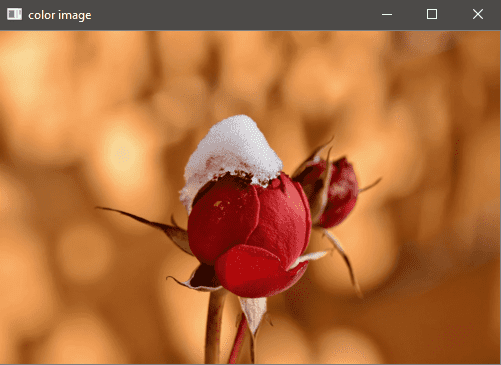
imshow()
function.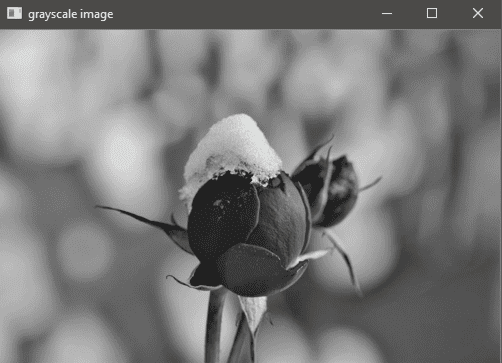
imshow()
function.
imshow()
function.The below GIF shows execution of the code to read and display the prototype but without waitKey()
. The window gets destroyed within milliseconds, and no output is shown on the screen.
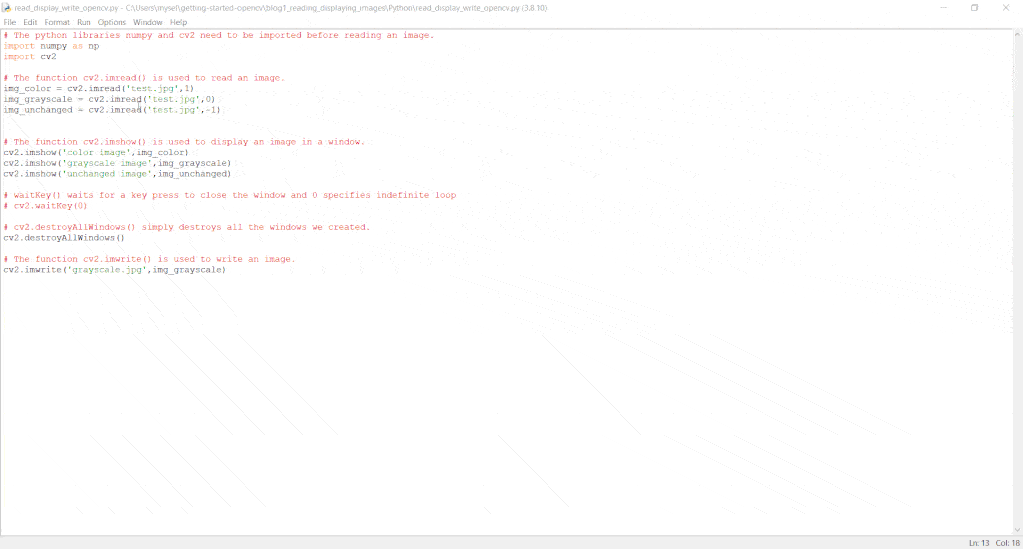
Writing an Epitome
Finally, let's discuss how to write/relieve an image into the file directory, using the imwrite()
function. Bank check out its syntax:
imwrite(filename, image)
.
- The offset argument is the filename, which must include the filename extension (for example .png, .jpg etc). OpenCV uses this filename extension to specify the format of the file.
- The second argument is the image you want to relieve. The part returns
Truthful
if the image is saved successfully.
Have a wait at the code below. See how unproblematic it is to write images to disk. Merely specify the filename with its proper extension (with any desired path prepended). Include the variable name that contains the paradigm data, and y'all're done.
Python
cv2.imwrite('grayscale.jpg',img_grayscale)
C++
imwrite("grayscale.jpg", img_grayscale);
Summary
Here, you learned to employ the:
-
imread()
,imshow()
andimwrite()
functions to read, display, and write images -
waitKey()
anddestroyAllWindows()
functions, forth with the display function to- close the image window on key press
- and clear any open image window from the memory.
You have to experiment a lot when it comes to the waitkey()
function, for it tin can exist quite disruptive. The more familiar yous get with information technology, the better you can use information technology. Do download the consummate lawmaking to get some easily-on experience. Practice well, as these are the basic building blocks that can actually assistance y'all learn and master the OpenCV library → OpenCV colab notebook.
Subscribe & Download Code
If y'all liked this article and would similar to download code (C++ and Python) and instance images used in this mail, please click here. Alternately, sign up to receive a costless Estimator Vision Resource Guide. In our newsletter, we share OpenCV tutorials and examples written in C++/Python, and Figurer Vision and Automobile Learning algorithms and news.
Source: https://learnopencv.com/read-display-and-write-an-image-using-opencv/
0 Response to "Read a Part of Image Using Opencv"
Postar um comentário